Introduction
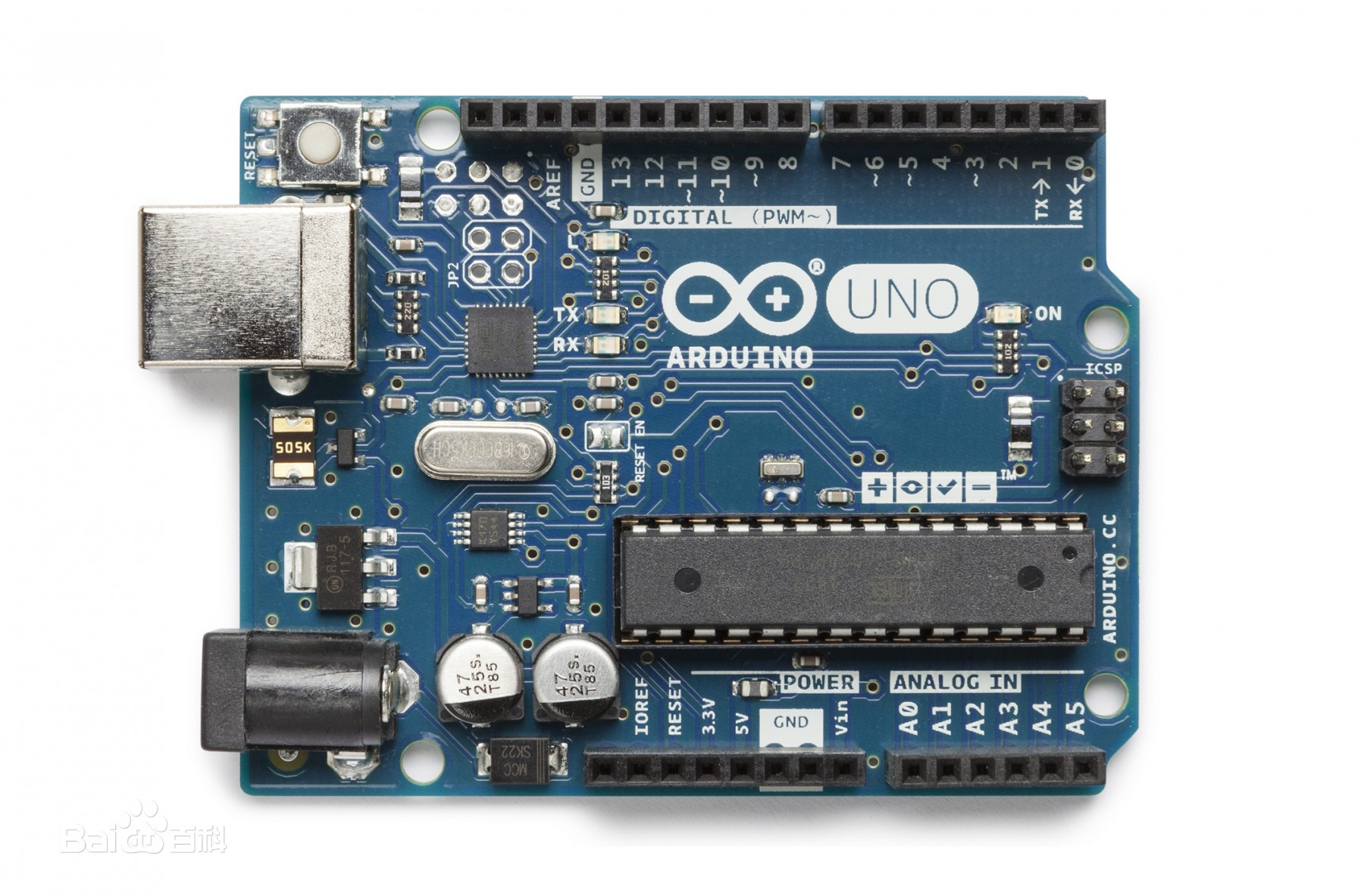
Arduino is a convenient, flexible, and easy-to-use open source electronic prototyping platform. It contains software (ArduinoIDE integrated development environment) and hardware (various models of Arduino boards). The hardware can be programmed by the software to sense and interact with the physical world.
The Arduino software program is created using the Arduino IDE, an integrated development environment on your computer. In the IDE, you can write and edit code and convert this code into instructions that the Arduino hardware understands, and then upload these instructions to the Arduino board, which enables you to control the Arduino board through the program.
Case 1
Human body pyroelectric sensor controls the traffic lights to change color.
The infrared digital signal is detected by the pyroelectric sensor of human body and converted into the digital signal of the traffic light through transmission, so as to control the color change of the traffic light.
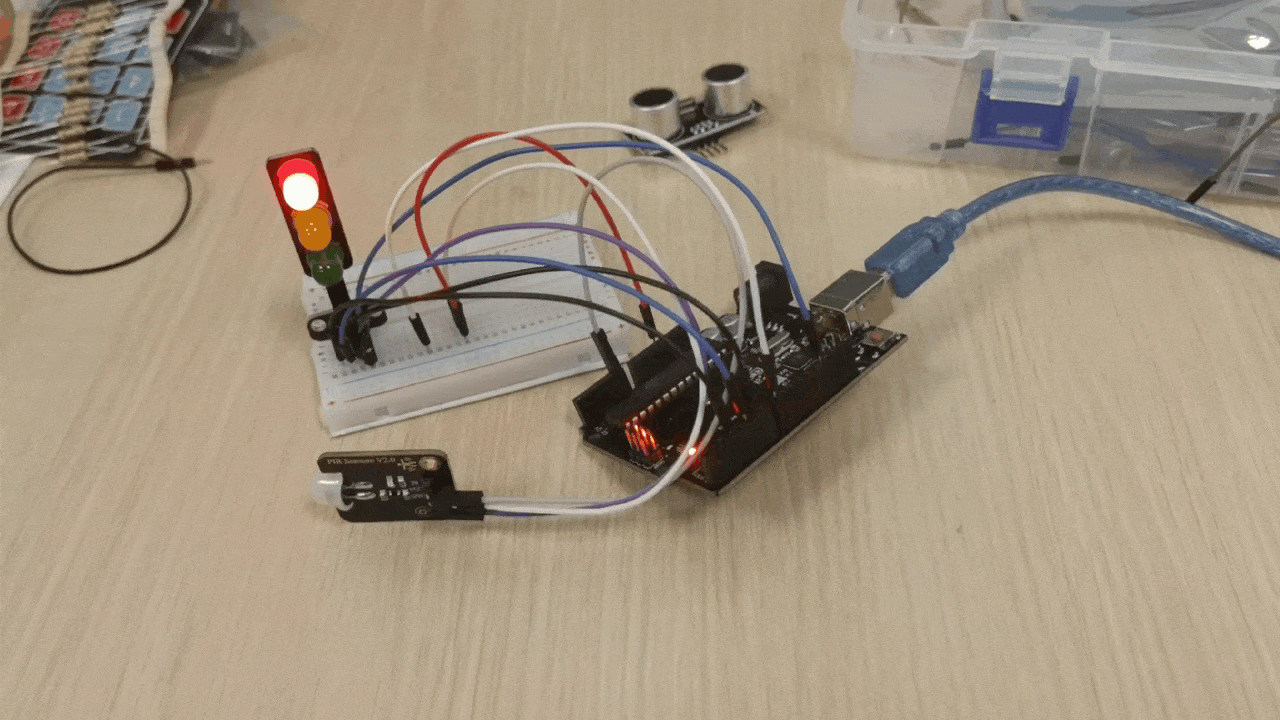
Selection of input module and output module
1.Human body pyroelectric sensor.
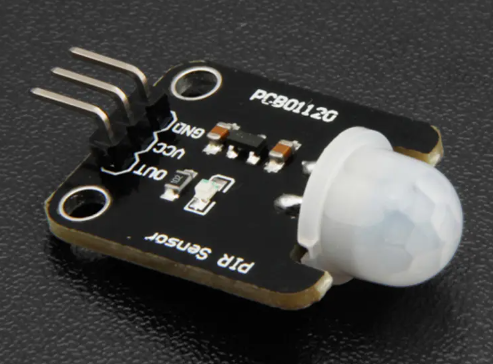
Pyroelectric infrared sensor is a kind of sensor that can detect infrared rays emitted by human or animal body and output electrical signals. As early as 1938, it was proposed to use pyroelectric effect to detect infrared radiation, but it was not taken seriously. It was not until the 1960s that the research on pyroelectric effect and the application of pyroelectric transistor were promoted with the rapid development of laser and infrared technology. Pyroelectric transistor has been widely used in infrared spectrometer, infrared remote sensing and thermal radiation detector, and it can be used as an ideal detector for infrared laser. Its goal is being widely used in various automatic control devices.
2.The traffic lights module
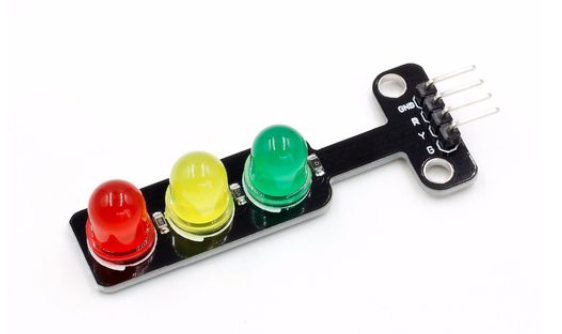
The traffic lights is consist of three colors led light, which is controled by 3 pins. It is the common module used in the arduino teching.
Tinkercad diagram
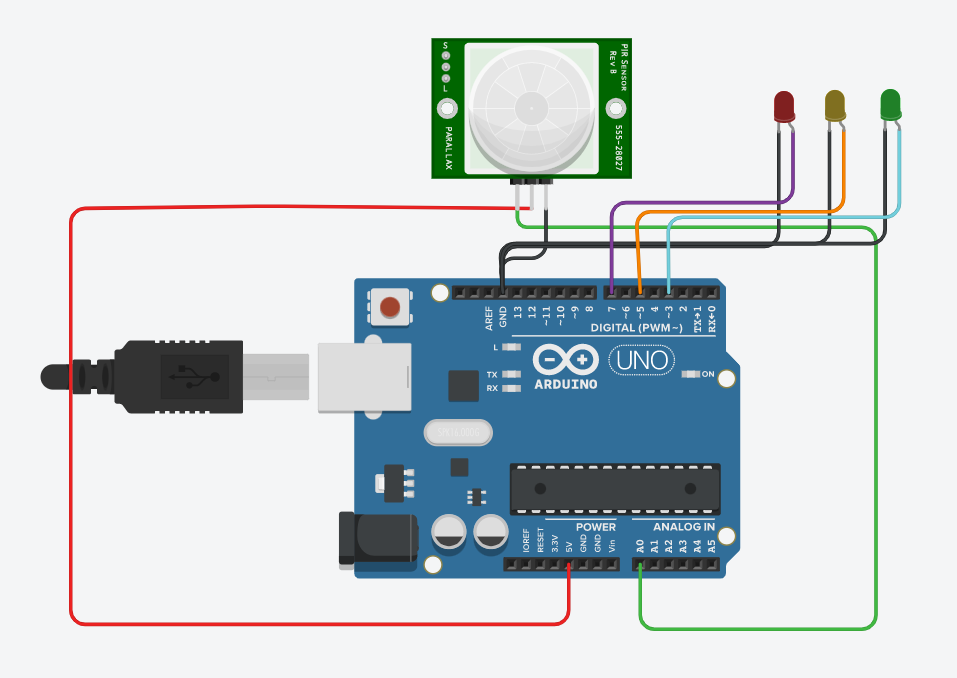
The code and basic logic of the case
The overall code logic is to control the light change of traffic lights through the high and low levels of human thermoelectricity sensors. When the sensor has a high signal (in the test, we found that the low signal value of the sensor is about 70, and the high signal value is about 700), the signal lamp will change color and light continuously for 5 seconds.
1 | dint red = 6; |
Case 2
Ultrasonic distance sensor controls the rotation angle of steering gear
In this case, the analog signal of ultrasonic wave is converted into a distance value, which is mapped to the angle of the steering gear, so that the rotation angle value of the steering gear is controlled by ultrasonic ranging.
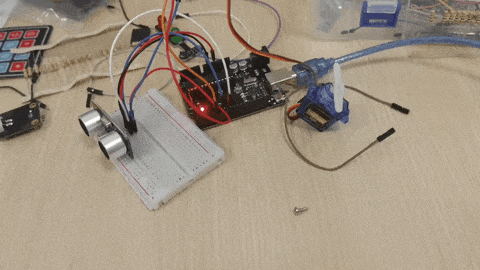
Selection of input module and output module
1.The tpye of ultrasonic distance sensor is HC-SR04.
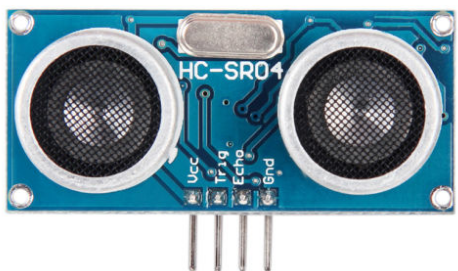
There are 4 pins in total, VCC can generally be connected to 3.3V-5V power supply, GND is grounded, and Trig is the trigger pin. IO is used to trigger ranging, and a low-high pulse is input to the Trig pin, and the high position is kept at least 10us. The module will automatically send 8 square waves of 40KHz to automatically detect whether there is a signal returning. When a signal returns, a high level is output through IO (specifically, the Echo pin), and the duration of the high level is read to get the time from the emission to the return of the ultrasonic wave.
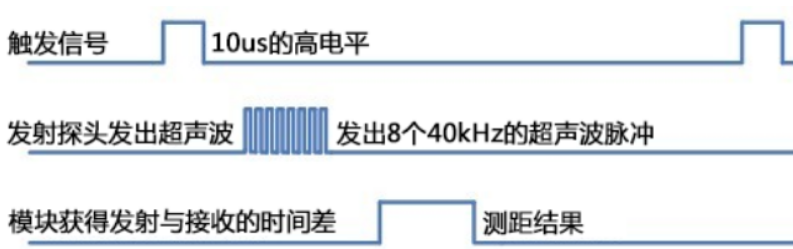
Through the above analysis, the test distance = (high level time * sound speed (343m/s))/2.
2.steering gear
Steering gear is a position (angle) servo driver. Steering gear is just a popular name, and its essence is a servo motor. It is widely used in control systems that need to keep the angle constantly changing. Such as remote control robots, airplane models, etc.
The rotation angle of the steering gear is 0 ~ 180, and its internal structure includes three parts: motor, control circuit and mechanical structure. The motor has three wires leading out, which are respectively connected with VCC, GNG and signal wire. There is main format of outgoing lines: Brown, red and orange (brown connected to GND, red connected to VCC and orange connected signal).
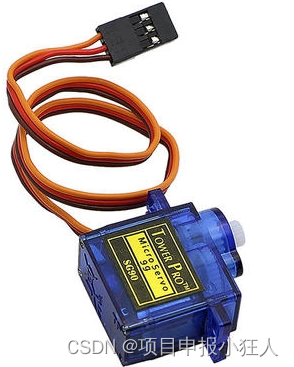
Tinkercad diagram
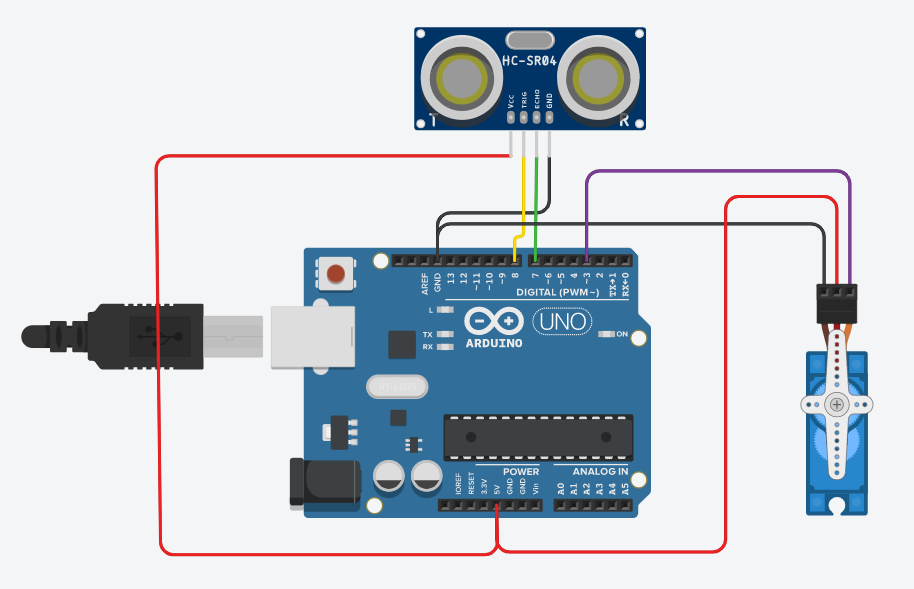
The code and basic logic of the case
The overall code logic is to control the light change of traffic lights through the high and low levels of human thermoelectricity sensors. When the sensor has a high signal (in the test, we found that the low signal value of the sensor is about 70, and the high signal value is about 700), the signal lamp will change color and light continuously for 5 seconds.
1 |
|
About this Post
This post is written by Guo Herui, licensed under CC BY-NC 4.0.