Introduction
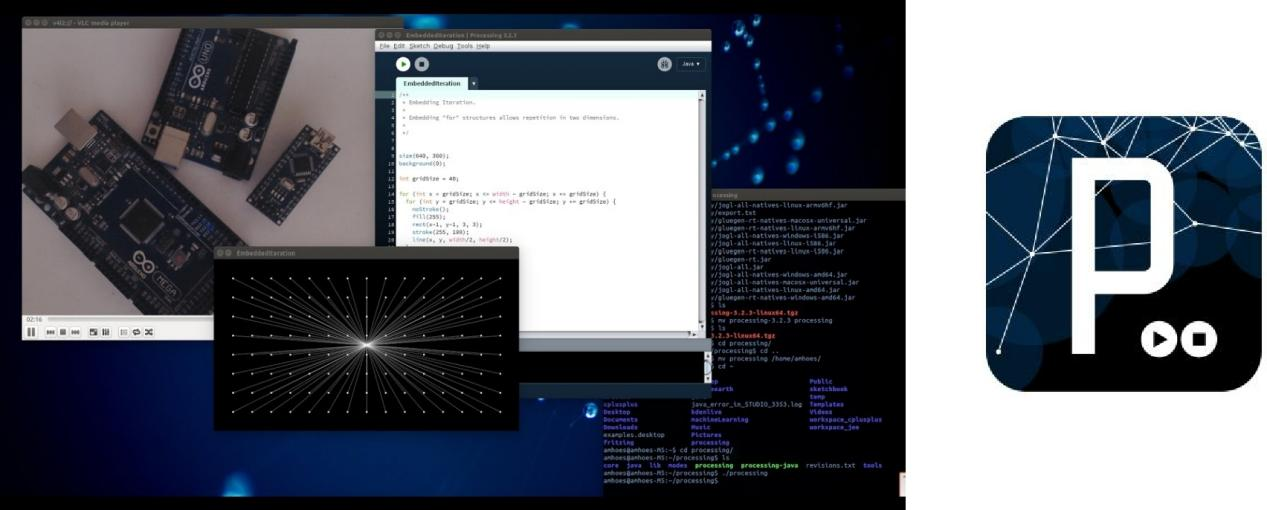
Processing is a flexible software sketchbook and a language for learning how to code. Since 2001, Processing has promoted software literacy within the visual arts and visual literacy within technology.
p5.js is a JavaScript library for creative coding, with a focus on making coding accessible and inclusive for artists, designers, educators, beginners, and anyone else! p5.js is free and open-source because we believe software, and the tools to learn it, should be accessible to everyone.
Using the metaphor of a sketch, p5.js has a full set of drawing functionality. However, you’re not limited to your drawing canvas. You can think of your whole browser page as your sketch, including HTML5 objects for text, input, video, webcam, and sound.
Our Work
Work 1
In the first work, we did one demo in Processing which can use mouse to interactive.
We have designed a pinball game, which named Rebound Ball. The player can use the mouse to click on the screen and the game will generate a small ball at the location where the player clicked and make it bounce in a random direction. When the generated ball collides with other balls on the screen, it will produce different collision states according to the kinematics principle. When the ball bounces to the border of the screen, it will also bounce back according to the kinematic principle.
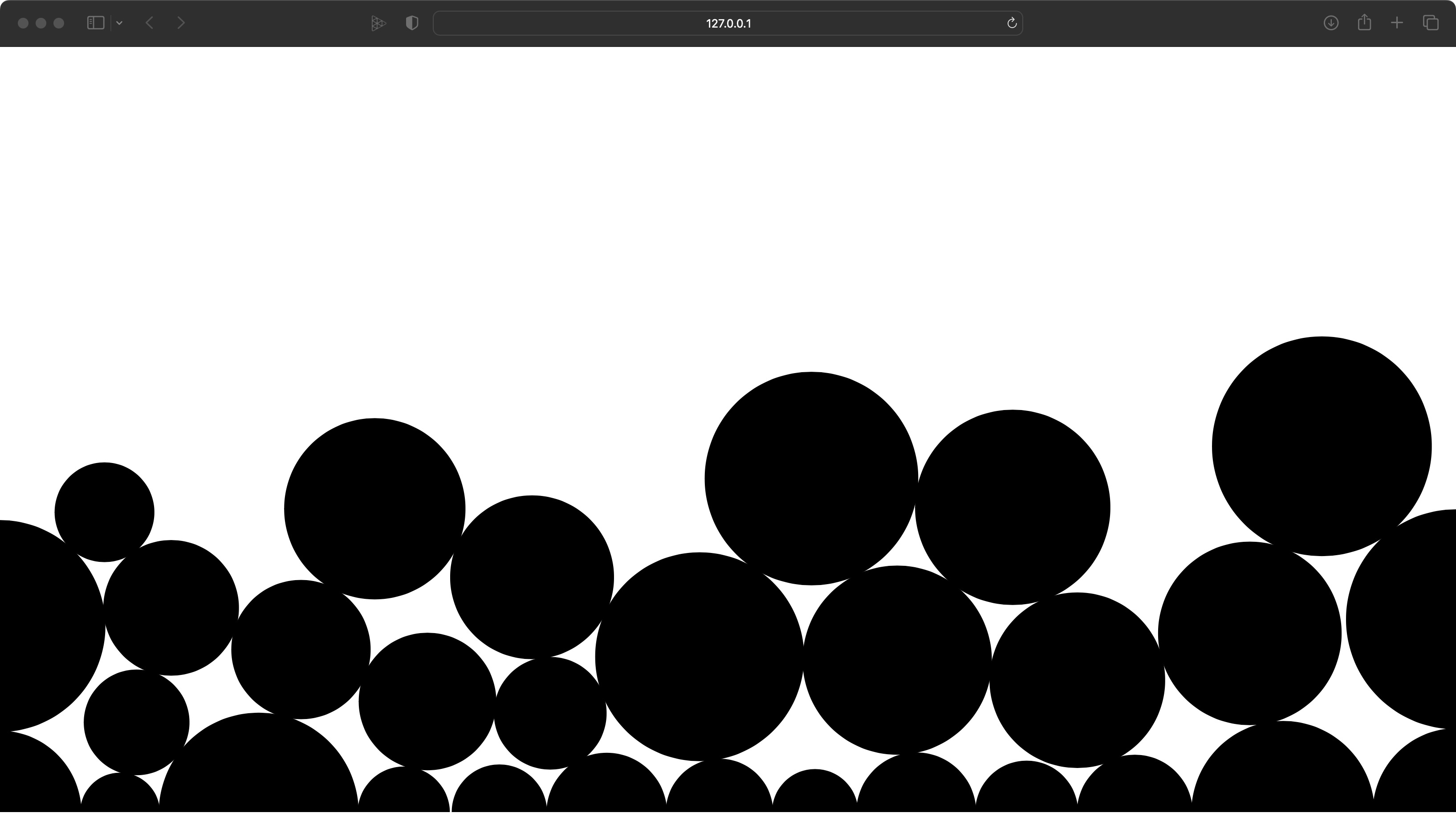
1.Install the p5.js language in Processing. Firstly, We have downloaded the p5.js library file from the official website. Secondly, Open the Processing software and import the p5.js library file into the Contribution Manager. Finally, adjust Processing’s input language to p5.js to program in that language.
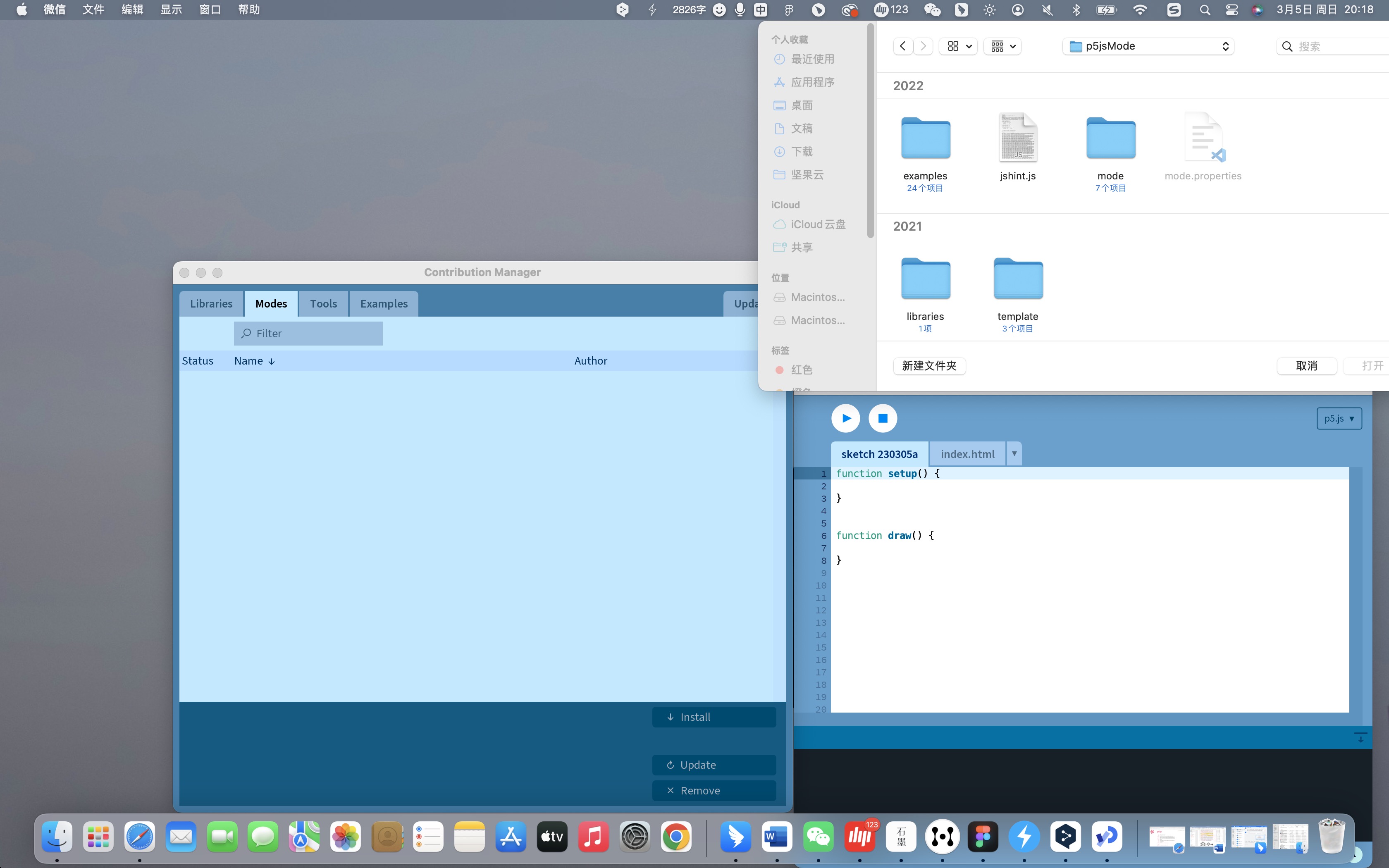
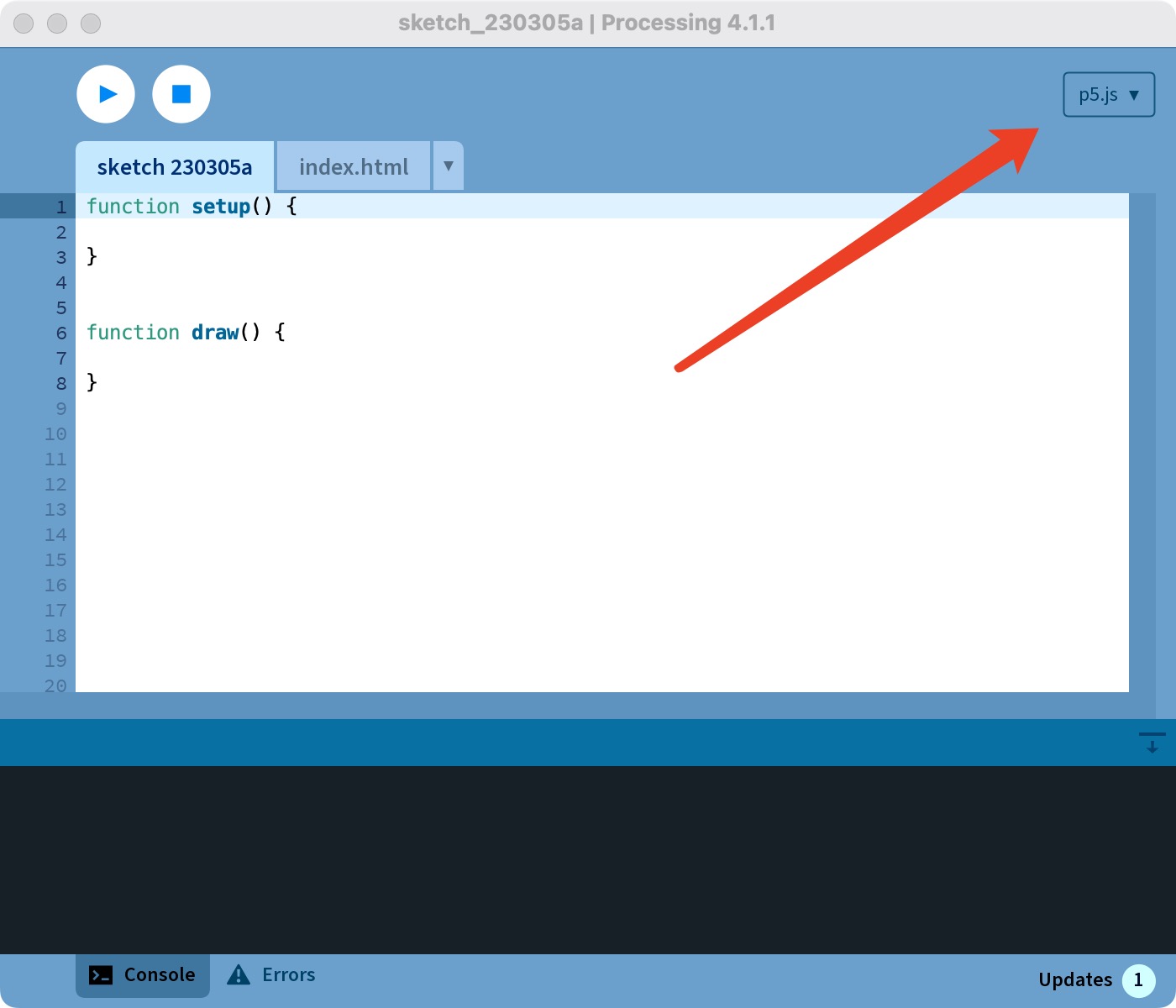
2.Write programs using the p5.js language. In the writing process, first define the upper limit of the number of balls in the game, then define the kinematic and mechanical environment of the game (including the properties of gravity, friction, etc.), and finally write the rules of the game.
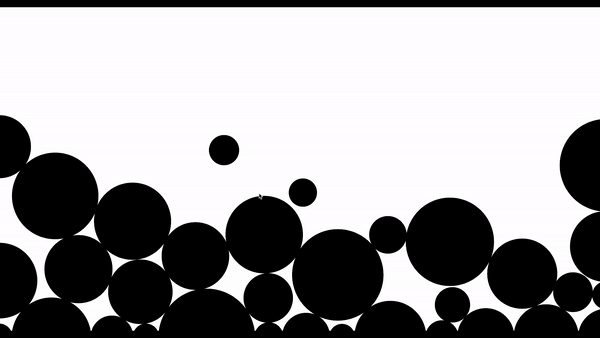
1 | let balls = []; |
Wrok 2
In the second work, we did one demo in Processing and Arduino, which can communicate with each other.
Human body pyroelectric sensor controls the movement of small square in Processing. This case is based on the pyroelectric sensor of human body, and the square in Processing is controlled to move left and right through arduino output signal, so as to realize the interaction between software.
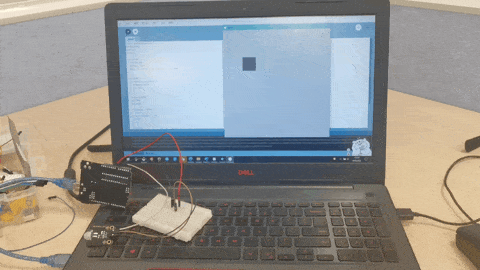
In arduino, the Gao Diping signal in human pyroelectric sensor is transmitted through serial port.
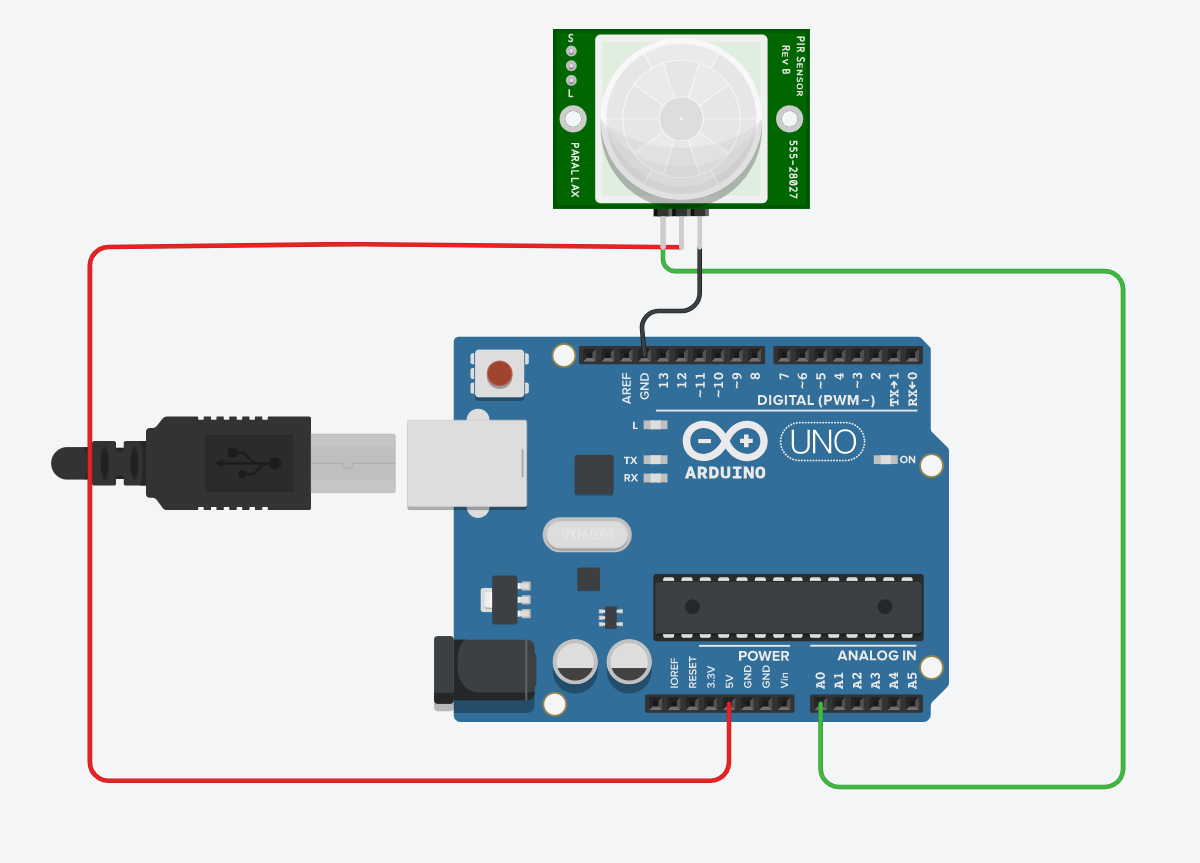
1 | int value = 0; |
Import the Serial library in Processing to accept the serial signal transmitted by arduino. Set the screen size and serial port information in setup. Then set the position of the square according to the signal and draw the square. In the actual picture, there will be an animation of the square moving.
1 | import processing.serial.*; |
About this Post
This post is written by Guo Herui, licensed under CC BY-NC 4.0.